Overview
TutorHelper is a desktop administration application intended for Tutors who would like to organise their students' information. TutorHelper uses a CLI (command-line interface) as its main form of input: tutors issue commands in the form of successive lines of text. Having multiple students at once can be a hassle to deal with, especially with each student having his / her own curriculum to learn, tuition timing, and payments to make. Our team of five decided to create TutorHelper by building upon the existing AddressBook Level 4 application. TutorHelper offers an easy way to keep track of your schedule and keep tabs on the students, allowing you to efficiently plan ahead. TutorHelper has a GUI created with JavaFX. It is written in Java, and has about 25 kLoC.
Some of the new main features include:
Grouping
Payment
Earnings
Add/Edit/Delete/Mark Syllabus
Add/Delete/Copy Subject
This project portfolio consists of the contributions I have made to TutorHelper.
Summary of Contributions
-
Contributed Code: RepoSense
-
Major Enhancement:
-
Added the ability to add and delete subjects taken to student entries (Pull Request #112)
-
This enhancement allows the tutor to add and delete subjects taken by their students. Each subject will have its own list of syllabus topics.
-
Justification: Students of tutors may request to receive tuition for more than one subject from the same tutor. This enhancement allows the tutor to record such instances instead of creating a new student entry for each subject.
-
Highlights: A student entry must have at least one subject taken at all times. Logically, a student entry should not exist if the student is not taking a subject taught by the tutor.
-
-
-
Minor Enhancements:
-
Other contributions:
-
Refactoring:
-
Project Management:
-
Managed releases v1.1 to v1.3.1 (4 releases) on GitHub
-
Created and labelled issues for the different milestones on Github
-
-
Enhancements to Existing Features:
-
Documentation:
-
Reviewed, formatted and standardised terminology in documentation (Pull Requests #47, #78, #87, #115, #159)
-
Added section for adding and deleting subjects in the User Guide
-
Added section for adding and deleting subjects in the Developer Guide
-
Re-ordered sections of User Guide for consistency (Pull Request #159)
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Add Subject : addsub
Adds a new subject to a specified student. Refer to valid subject inputs under 3. Feature.
Format: addsub STUDENT_INDEX s/SUBJECT
|
Examples:
-
`addsub 1 s/Physics
Adds a subject "Physics" for the first student. -
`addsub 2 s/Mathematics
Adds a subject "Mathematics" for the second student.
Delete Subject : deletesub
Deletes a subject from a specified student.
Format: deletesub STUDENT_INDEX SUBJECT_INDEX
Attempting to delete the only subject left for a student will throw an error. A student must have at least one subject. |
Examples:
-
deletesub 1 2
Deletes the second subject from the first student. -
deletesub 3 1
Deletes the first subject from the third student.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Add / Delete Subject Feature
Implementation
The add / delete subject mechanism is facilitated with TutorHelperParser
.
Classes related to the functionality of the mechanism are listed below:
-
TutorHelperParser
— Creates aAddSubCommandParser
/DeleteSubCommandParser
object and parses the user’s input. -
AddSubCommandParser
/DeleteSubCommandParser
— Analyses user input to create a correspondingCommand
object. -
AddSubCommand
/DeleteSubCommand
— Execution results in addition / deletion of a subject for a specified student index.
Given below is an example usage scenario of how the add / delete subject mechanism behaves at each step.
Add Subject
Step 1. The user launches the application.
Step 2. The tutor executes a command of the format addsub STUDENT_INDEX s/SUBJECT
on the CLI.
Step 3. The arguments are parsed by AddSubCommandParser
, which produces an instance of AddSubCommand
.
Step 4. AddSubCommand.execute()
is called, and the supplied subject is added for the student at the specified student index by TutorHelper.
Delete Subject
Step 1. The user launches the application.
Step 2. The tutor executes a command of the format deletesub STUDENT_INDEX SUBJECT_INDEX
on the CLI.
Step 3. The arguments are parsed by DeleteSubCommandParser
, which produces an instance of DeleteSubCommand
.
Step 4. DeleteSubCommand.execute()
is called, and the subject at the specified subject index of the student at the specified student index is deleted by TutorHelper.
The TutorHelper has to have at least 1 student as a precondition for both addsub and deletesub .
|
deletesub requires the student at the specified student index to have at least two subjects. After deletion, a student cannot have 0 subjects.
|
The following sequence diagram shows how the addsub
operation works:
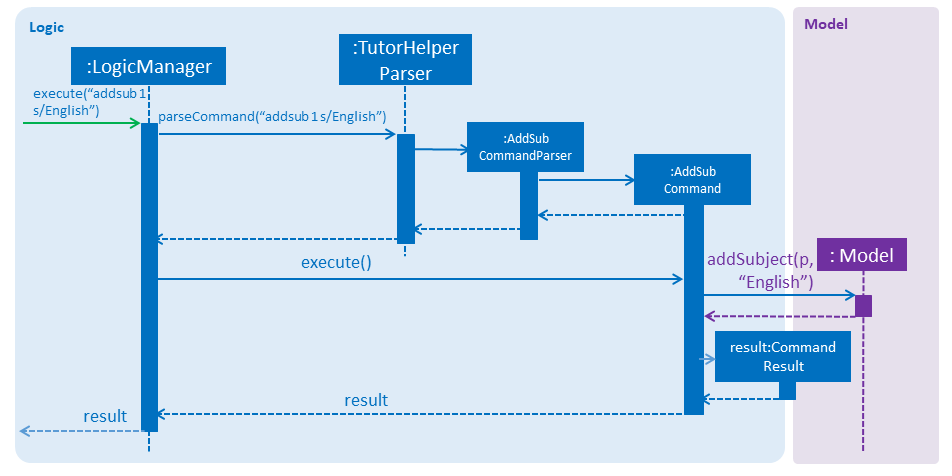
The following sequence diagram shows how the deletesub
operation works:
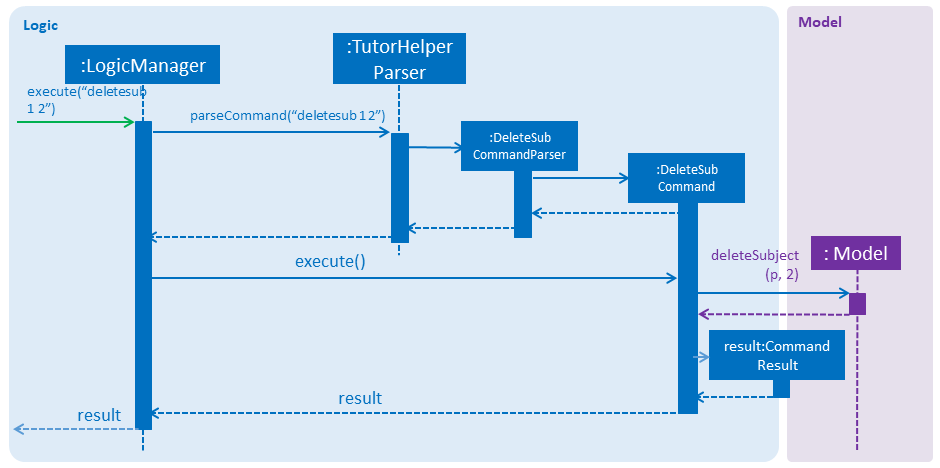
User Stories
-
As a busy tutor, I want to be able to manage my students' schedules individually, so that I can plan my time properly.
-
As a tutor, I want to be able to find out where my students live and what time I should be there for tuition.
-
As a tutor, I want to know my students' individual progress so that I know what topics I need to cover for the next tuition session.
-
As a tutor, I want to keep track of my students' payments so that i know who to collect fees from.
-
As a tutor, I want to keep track of my monthly earnings so that i can manage my financial accounts.
-
As a tutor, I want to be able to edit teaching data such as editing my syllabus to keep up with changes in school’s curriculum or updating payments made by students.
Use Cases
-
Add Student
System: TutorHelper Actor: Tutor MSS: 1. Tutor inputs to add a student and his/her details. 2. System adds student details into the database. Use case ends. Extensions: 2a. Tutor did not key in all mandatory fields. 2a1. System displays error message informing tutor of invalid index. 2b1. Resume step 1.
-
Edit Student
System: TutorHelper Actor: Tutor MSS: 1. Tutor inputs to edit a student's details. 2. System edits student details into the database. Use case ends. Extensions: 2a. Index is out of bounds 2a1. System displays error message informing tutor of invalid index. 2b1. Resume step 1.
-
Delete Student
System: TutorHelper Actor: Tutor MSS: 1. Tutor inputs student's index to delete. 2. System deletes student details from the database. Use case ends. Extensions: 2a. Index is out of bounds 2a1. System displays error message informing tutor of invalid index. 2b1. Resume step 1.
-
List Students
System: TutorHelper Actor: Tutor MSS: 1. Tutor requests to list students. 2. System displays current list of students. Use case ends.
-
Group Students
System: TutorHelper Actor: Tutor Precondition: Current list of students is not empty. MSS: 1. Tutor requests to group students by day or timing. 2. System filters the current list of students based on the timing entered. 3. System sorts the filtered list of students in order of timing. 4. System displays filtered and sorted list to Tutor. Use case ends. Extensions: 2a. Input is invalid 2a1. System displays examples of valid input to Tutor. Use case ends.
-
Record students' payments
System: TutorHelper Actor: Tutor Precondition: Current list of students is not empty. MSS: 1. Tutor request to add in payment for a student. 2. System searches for that student according to index entered. 3. System adds Payment amount, month and year to student's record. 4. System displays payment record in browser panel. 5. System displays successful recording of payment message under command box. Use case ends. Extensions: 2a. Tutor does not enter all the required entries correctly 2a1. System displays error message 2a2. System gives tutor an example of a correct entry. 2a3. Repeat step 1 2b. Tutor does not enter a valid student index 2b1. System displays error message telling tutor that index is invalid 2b2. Repeat step 1.
-
Edit students' payments
System: TutorHelper Actor: Tutor Precondition: Current list of students is not empty. Precondition: Payment for the month and year has been recorded for student before. MSS: 1. Tutor request to add in edited payment for a student. 2. System finds the existing payment entry with same month and year. 3. System adds new payment entry to existing entry. 4. System displays payment record in browser panel. 5. System displays successful editing of payment message under command box. Use case ends. Extensions: 2a. Tutor does not enter all the required entries correctly 2a1. System displays error message 2a2. System gives tutor an example of a correct entry. 2a3. Repeat step 1 2b. Tutor does not enter a valid student index 2b1. System displays error message telling tutor that index is invalid 2b2. Repeat step 1.
-
Display tutor’s earnings for that month and year
System: TutorHelper Actor: Tutor Precondition: Current list of students is not empty. MSS: 1. Tutor request for earnings for a specific month and year. 2. System searches for all the payment records made from all the students for that particular month and year. 3. System adds up all the payment. 4. System displays total earnings under the command box. Use case end. Extensions: 2a. System does not find any payment recorded for that month and year. 2a1. System displays $0 as result. 2b. Tutor does not enter all the required entries correctly 2b1. System displays error message 2b2. System gives tutor an example of a correct entry. 2b3. Repeat step 1.
-
Edit students' syllabus for that subject
System: TutorHelper Actor: Tutor Precondition: Tutor has an existing syllabus entry at the index. MSS: 1. Tutor request to edit syllabus for a specific student, subject and syllabus 2. System searches for the student and the respective subject and syllabus at specified index. 3. System edits the syllabus at the specified index with new syllabus 4. System displays edited syllabus list in browser panel 5. System displays successful editing of syllabus under command box. Use case end. Extensions: 2a. System does not find any student entry at specified index. 2a1. System displays error message telling tutor that index is invalid 2a2. Repeat step 1. 2b. System does not find any subject entry at specified index. 2b1. System displays error message telling tutor that index is invalid 2b2. Repeat step 1. 2c. System does not find any syllabus entry at specified index. 2c1. System displays error message telling tutor that index is invalid. 2c2. Repeat step 1. 2d. System finds same syllabus entry already exists in subject. 2d1. System displays error message telling tutor that syllabus already exist. 2d2. Repeat step 1.
Non Functional Requirements
-
The system should respond in two seconds.
-
The system should be understandable to a novice in working with computers.
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 students without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.